Given an array of integers and a target value, return the indices of 2 numbers that add up to the input target value.
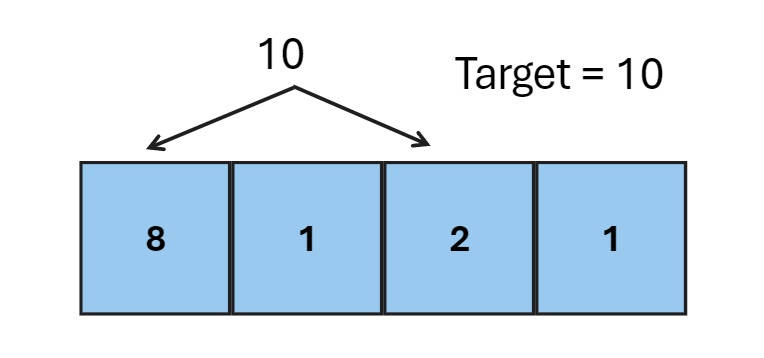
The first “two sum” in our example is 8 and 2, because they add up to 10.
LeetCode also asks us to return the indices which are [0, 2]
- There cannot be multiple solutions and only one solution will be found.
- You will also be guaranteed a solution.
- Only distinct numbers will be accepted (
[2,2]
are not valid pairs.
Hash Map Solution
The most common solution would be to declare two pointers and search for pairs that equal the target.
- First, initialize a pointer at the first position. Start a loop with p1 as our loop’s index from 0 till end of array.
- The second value we are searching for must be equal to the target minus the first pointer
- Search each remaining value for the target minus the first pointer
- Lastly, most first pointer and repeat.
twoSum(numbers, target) {
let indices = {};
for(i=0;i<numbers.length;i++){
var num = target - numbers[i]
if(indices[num] !== undefined) {
return [indices[num], i]
}
indices[numbers[i]] = i;
}
}