Array swapping is an algorithm pattern that is used constantly in a variety of interview questions and sorting algorithms. To put in simply, array swapping is just switching two array elements. In our example, we have an array of numbers. We want to swap the element at index 1 (which…
How To Capitalize Words In A Sentence (JavaScript)
Believe it or not, there is no magical method to capitalize words in a string. Not only is this a very common algorithm, but it is a common task. Just like the words in the title of this blog, sometimes capitalizing each word makes things look good! Using charAt() Happy…
Algorithm Patterns 101: Reversing Linked List
Linked List is a data structure which stores the data in a linear way. Unlike an array, data in a linked-list is non-contiguous (aka the data does not exist next to each other). Each element of a linked list contains a data field to store the list data and a…
Algorithm Patterns 101: Array Chunking
“Chunk” is one of the coolest words in the English language. Chunking is also a very useful algorithm technique where we split arrays into separate parts. Although there are many way to split an array, we are going to use a traditional for loop. Our pseudo code will look something…
Algorithm Patterns 101: Character Mapping
You will often be ask questions in interview settings that involve finding patterns within strings. In order to correctly track and correlate where characters exist, we need to use something called a character map. In this scenario, I am attempting to turn my name “Teddy” into a char map. While…
Algorithm Patterns 101: Sliding Window
The sliding window pattern is used to process sequential data by maintaining a moving subset of elements. When we first start our algorithm journey our first inclination is to use nested loops. Sliding window is aimed at reducing our habit of using nested loops. A “window” is a data structure…
Algorithm Patterns 101: Fast and Slow Pointers
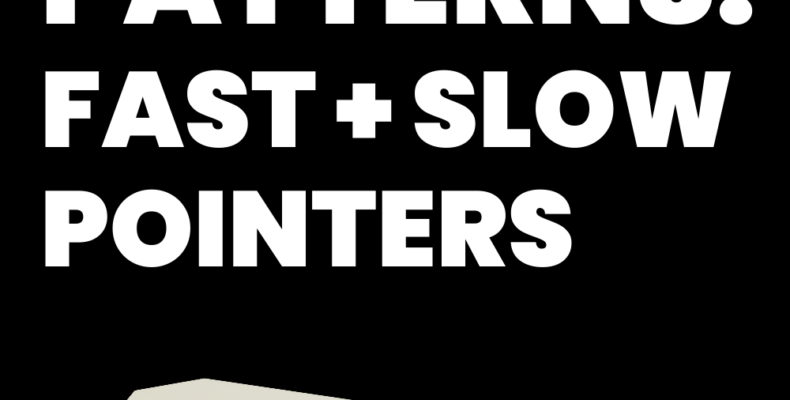
Similar to two pointers, fast and slow pointers use two pointers to traverse data structures. But this comes with one key difference, the pointers iterate at different speeds. The pointers can be used to traverse the array or list in either direct, BUT one must move faster than the other….
Algorithm Patterns 101: Two-Pointer
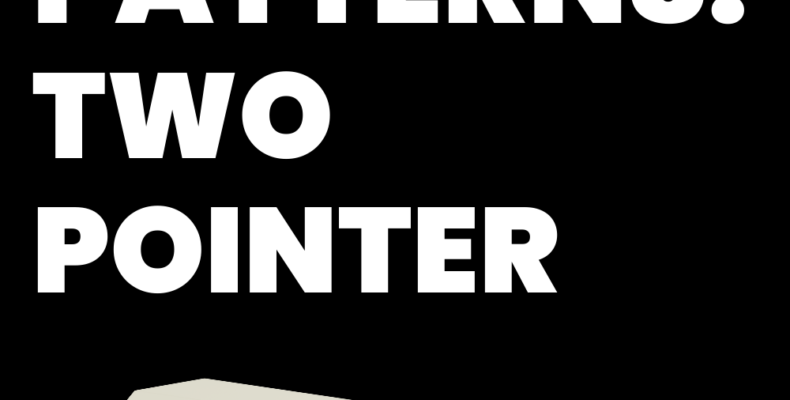
Two pointers is simply an alogorithm pattern that uses multiple pointers to iterate over a collection. What is a “pointer”? A pointer is just a number that stores where you are at. Almost like a bookmark, it keeps track and indexes the current place you are iterating. Why do we…