Software development (and more in particular front end development!) is an art form. We want our code to be beautiful and to a certain extent we are expressing our emotions (you know the usual, fear, anger, and unrestrained greed lol). The computer needs to “do something” or react to our code. In order for this to happen, we need a canvas and brush. In JS, the Execution Context is our canvas and the global object is our paint.
What is the Execution Context?
Ask this answer and you will receive many interpretations, but an execution context is simply the environment in which your code is running!
Before you write any code in JS, 2 important things are created for you:
- Global Object (aka ‘window’ object)
This global object can easily be seen and manipulated by typing in “window” into the Chrome Developer tools console.
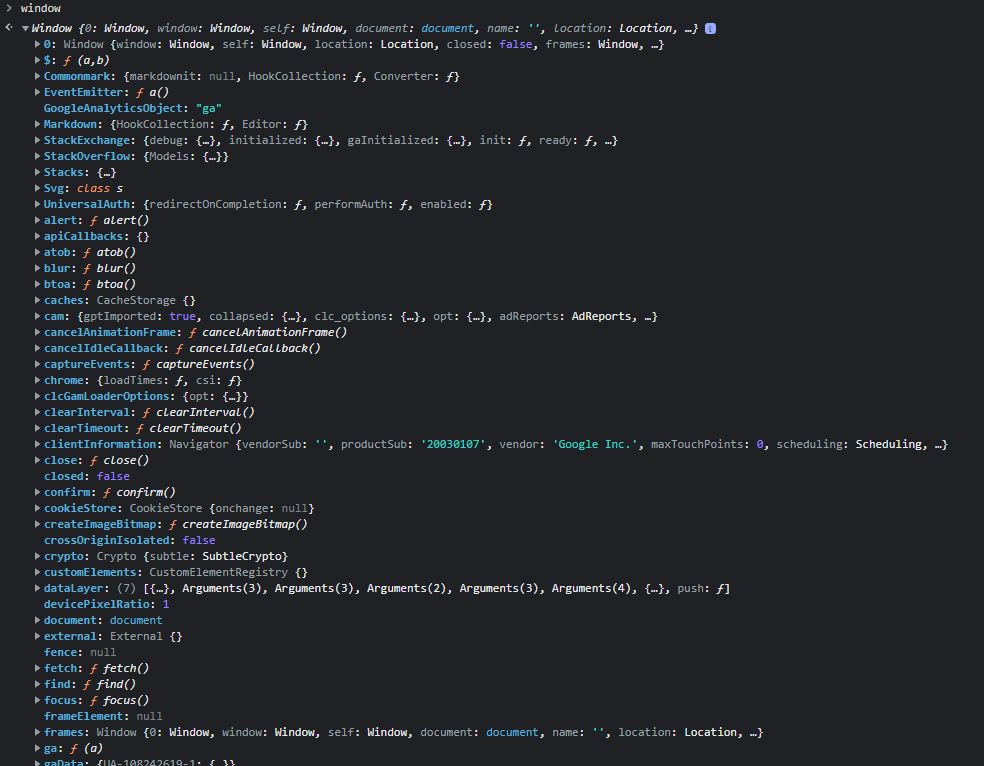
This giant object is essentially represents the browser and allows you to manipulate the browser to do anything you like.
2. “this” keyword is created and assigned to Global Object
“this” keyword is by far one of the most confusing topics in software developer, but it can easily be understood by learning the origins. When the execution context is first created the global “window” object is made assigned to “this”.
Essentially, the global object and “this” keyword are the same thing (until you create another execution context but let’s not get ahead of ourselves). Type in “this” keyword into Chrome Developer Tools console and you will see the same values as the global object.
Creation Phase
Let’s see how JS creates an Execution by considering this scenario:
b();
console.log(a);
var a = "Hello World!";
function b(){
console.log("Called b!");
}
The code above will run BUT it begs the question: Why will b() run even though the actual function to create comes after the execution of the code? Because the execution context starts working before you run the code! It parses and looks thru everything looking for variables and functions to store. You can learn more about parsing in my other article JavaScript Engine Explained!
This parsing process is called “hoisting” and is the reason functions can be executed BEFORE they are declared. Another important thing to remember, is that all variables are assigned undefined.
Execution Phase
After the creation phase, JS enters what is known as Execution Phase. Since JavaScript is single threaded it executes code line by line and creates new execution context for each function.
The function (along with the newly created execution context) is placed on top of the Global Execution context created at first.
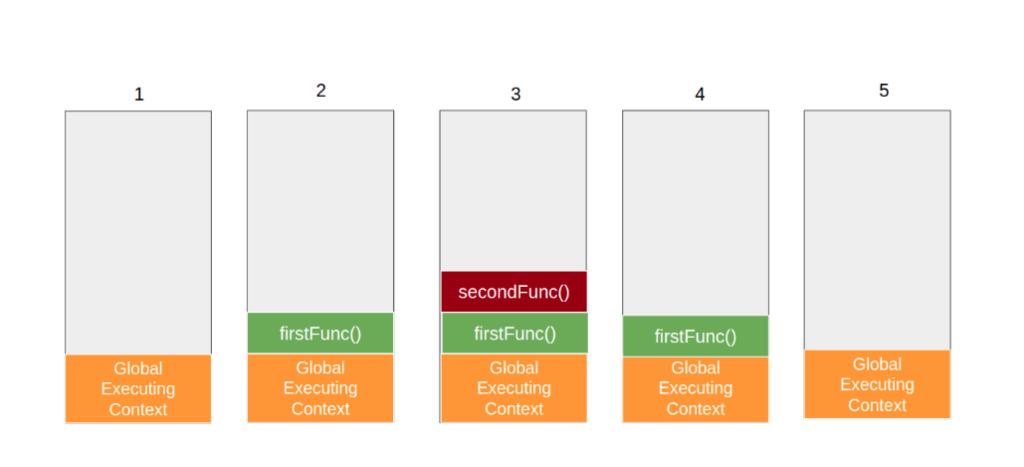