Learning closures and how they work under the hood will greatly increase your ability to write JavaScript code.
Before learning closures, it is important to have a good understanding of scope.
What is Scope?
Scope determines the accessibility or visibility of variables in JavaScript. There are three types of scope in JS are Global, Function, and Block:
- Global Scope
- Function Scope
- Block Scope
When you first being coding JS (and haven’t started on functions), this is likely what scope your code resides in. Essentially, any code outside a function will likely reside in Global Scope.
An easy way to understand scope is to just remember “global” scope can be accessed anywhere, while function and block scope cannot. Function scope simply means, code within a function cannot be “seen” outside. Block scope is any other code that is within {} brackets like if statements and while loops.
You may be asking yourself, well why would we need to isolate our variables within different scopes? Scope provides:
- The ability to isolate variables so that they don’t have naming collisions.
- Having tons of global variables will inevitably lead to performance & readability issues
- Allows each function to have it’s own self-contained “state”. (State, in this case, is a fancy word for “variables that change often”)
Scope Nesting
Before learning closure, it is also important to understand scope nesting and exactly how variables can be accessed.
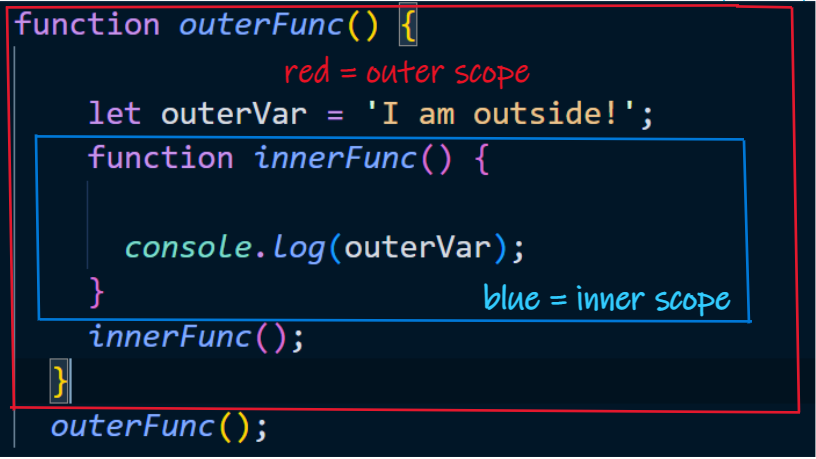
Notice the outerVar variable can be accessed inside the innerFunc(). The variables of the outer scope are also accessible inside the inner scope.
Important things to understand about the picture above:
- Scopes can be nested inside other functions
- The variable of the outer scope is accessible inside the inner scope.
What is Closure?
A closure in JavaScript is a function that has access to the outer (enclosing) function’s variables even after the outer function has returned. This is possible because the closure maintains a reference to the outer function’s variables, allowing them to remain in memory even after the outer function has finished executing.
One common use of closures in JavaScript is to create private variables that can only be accessed by the inner function. For example:
function createCounter() {
let count = 0;
return function() {
count++;
return count;
}
}
console.log(createCounter()); // 1
console.log(createCounter()); // 2
In the above example, the inner function has access to the count variable even after the createCounter function has returned. This allows the counter function to maintain its own private state and increment the count variable each time it is called.
This is useful for creating functions that can be called at a later time, and maintain their own state. It is also commonly used in event handlers and other asynchronous code, where the inner function needs to have access to variables from the outer scope.