An iterator is simply an object that is used to traverse a data structure. Unless you are working on an old Java code base, iterator patterns are going to be few and far between.
Although rare, many of the features we take for granted exist because of this pattern. It’s incredibly useful to understand how things work! (no suprise)
But why? The idea in this pattern is:
- Create your own data structure
- Make methods that allow you to access, traverse, and index.
- Encapsulate so that you can do all this with just methods
Iterators are everywhere
foreach(x in y) {
Console.WriteLine(x);
}
Anytime you see code like this, iterators are at work. The compiler is actually translating this code to:
var enumerator = ((IEnumerable<Foo>)y).GetEnumerator();
while (enumerator.MoveNext())
{
temp = enumerator.Current;
Console.WriteLine(temp);
}
Although this is C# code, foreach
exists in nearly all languages and makes life easier. No more having to code out variables to place in a regular for
.
Making a simple iterator
Let’s say we want to create a simple iterator for integers:
To make an iterator we must first create an interface with they type of iterations we want.
public interface Iterator {
boolean hasNext();
int next();
}
Next, we implement a concrete example:
public class IntegerIterator implements Iterator {
int[] items;
int position = 0;
public IntegerIterator(int[] items) {
this.items = items;
}
public int next() {
int[] user = items[position];
position = position + 1;
return user;
}
public boolean isEmpty() {
if(position >= items.length || items[position] == null) {
return true;
} else {
return false;
}
}
}
Now, we create the iterator:
public static void main(String[] args) {
int numberArray = new { 1, 2, 3, 4, 5 };
IntegerIterator interator = new Integeriterator(numberArray);
while(!iterator.isEmpty()) {
System.out.println(iterator.next());
}
System.out.println("Done.");
}
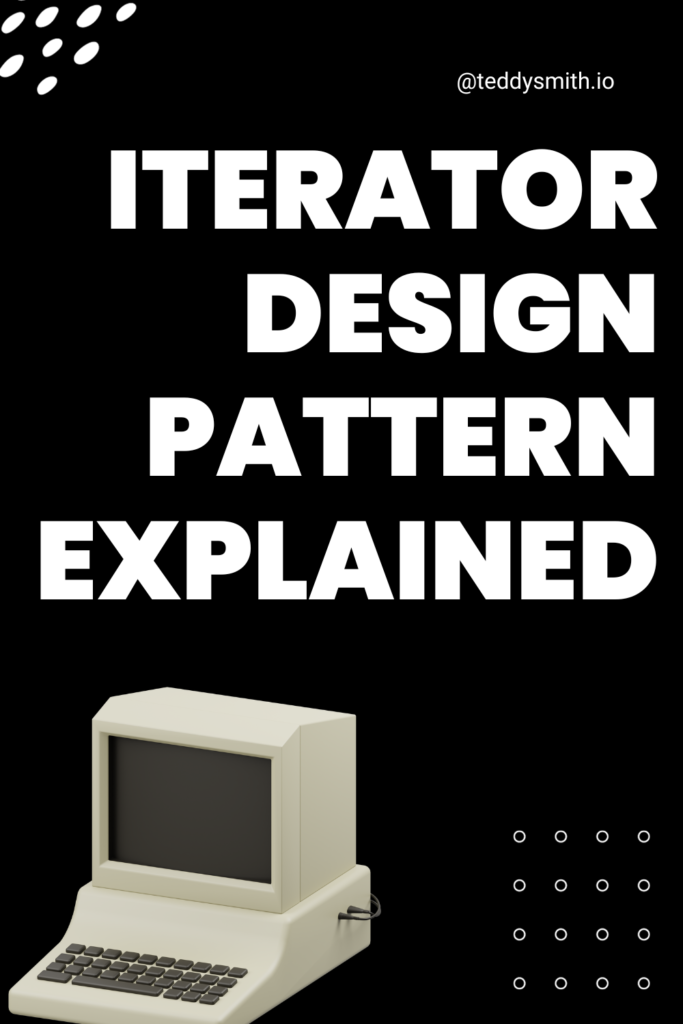