JavaScript is a weird language. We are all aware of this. But one of the biggest peculiarities is the concept of hoisting. Because hoisting is centered around variable declaration, it confuses beginners to no end. In this blog, I am going to give practical, real-world explanations of hoisting!
Hoisting Introduction
Hoisting is a default behavior which variables and function are moved to the top of the scope before JS executes. Even more simply put, it allows you to use variables and functions before declaring them.
x = 1;
console.log(x); //This is valid and will execute.
var x;
Essentially, JavaScript is taking “var x;” variable declaration and placing it above x = 1.
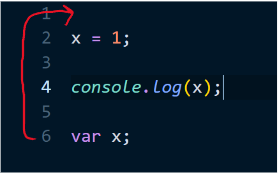
The biggest caveat to hoisting is that it only works with declaration BUT not initialization of variables.
console.log(x) //This will be undefined because it is being initialized.
var x = 1;
Hoisting Functions in Javascript
JavaScript engine moves function declarations to the top similar to variables.
console.log(sayHello()); //console logs "hello"
function sayHello(value1, value2) {
return "hello";
}
Very similar to variable initializations, the JavaScript engine DOES NOT move a function expression;
sayHello(); // JS return "sayHello not defined"
var hello = function sayHello() {
console.log("hello");
}
Hoisting Variables Before Functions
The last hoisting concept to understand is that functions are declared before variable declarations.
console.log(sayHello); // Javascript will return [function] or the actual function
}
var sayHello;
function sayHello() {
console.log("say hello");
}
In the example above, the JavaScript compiler will return the actual function because it is moves before the variables.