Array swapping is an algorithm pattern that is used constantly in a variety of interview questions and sorting algorithms.
To put in simply, array swapping is just switching two array elements.
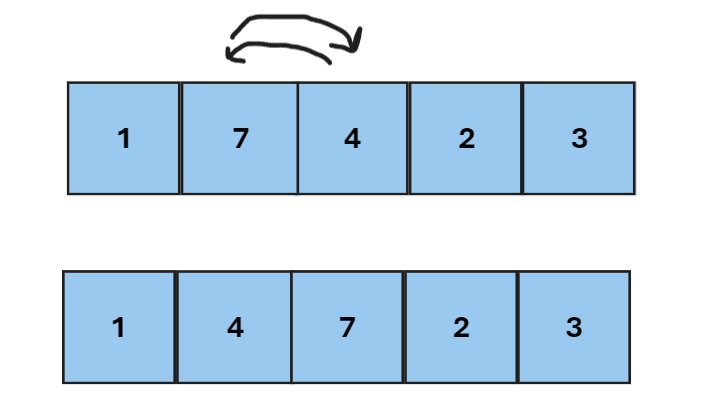
In our example, we have an array of numbers. We want to swap the element at index 1
(which is 7), with the element at index 2
(which is 4). There are many ways to make this happen and this blog will teach you two ways:
Temp Variable Array Swap
In this example, let’s start with something easy by simply swapping the first and second elements.
- First, we temporarily store the contents of one of the items we want to swap. This could be either the first or second item, but let’s choose the first because its easy.
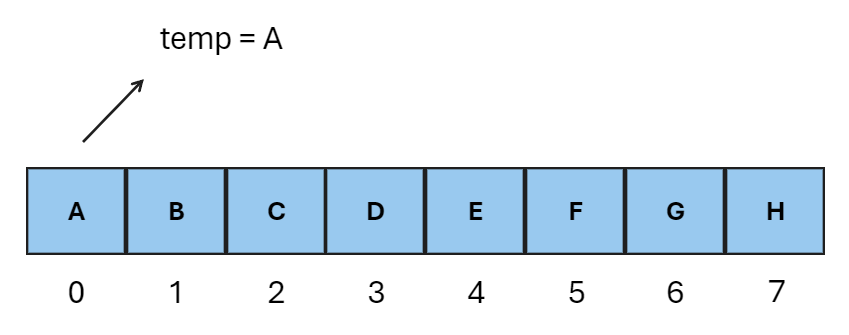
- Second, with the contents of the first item stored for safe keeping, we then assign the context of the second item to the first item.
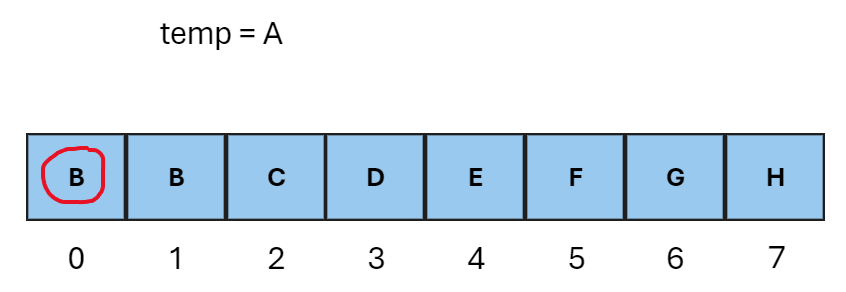
- Third, set the value of the second element to the value in the temp variable.
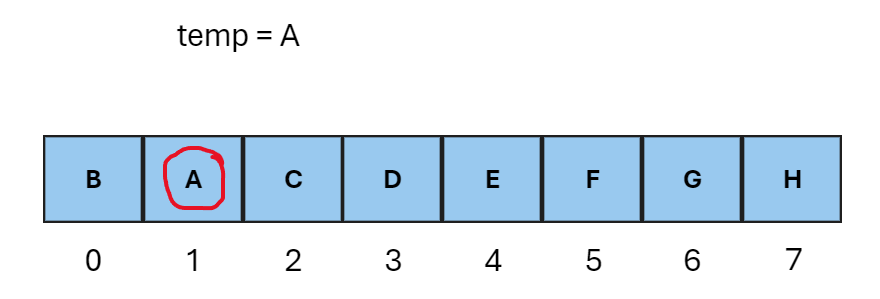
//Set temp to first index
//Set what we just "temped" to the value that you want to change
arr[0] = arr[1];
//Set the second value to the temp value
arr[1] = temp;
We code hard code our data into the array accessors, but it is way easier to just make a function to be able to plug in our numbers.
const swapElements = (arr, index1, index2) => {
let temp = arr[index1];
arr[index1] = arr[index2];
arr[index2] = temp;
}
Arrays Swap Destrucuring
A far more aesthetic way to array swap is destructuring.
With destructuring, you can use a simple one-liner to perform an array swap like this:
[arr[0], arr[1]] = [arr[1], [arr[0]];
Once again, we hardly ever want to hard code values, so we create a simple swap function to handle it for us:
const swapElements = (arr, index1, index2) => {
[arr[index1], arr[index2]] = [arr[index2], [arr[index1]];
};
Conclusion
In this blog post, we learned an important algorithm technique to swap array values! Happy coding!