“Chunk” is one of the coolest words in the English language. Chunking is also a very useful algorithm technique where we split arrays into separate parts.
chunk([1, 2, 3, 4], 2) --> [[1, 2], [3, 4]]
Although there are many way to split an array, we are going to use a traditional for loop. Our pseudo code will look something like this:
Step-By-Step
- Determine the chunk size
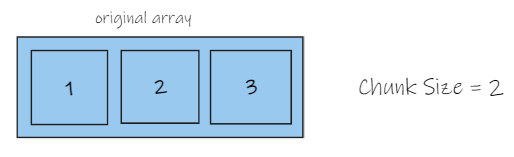
- Create a new array to put our chunks in
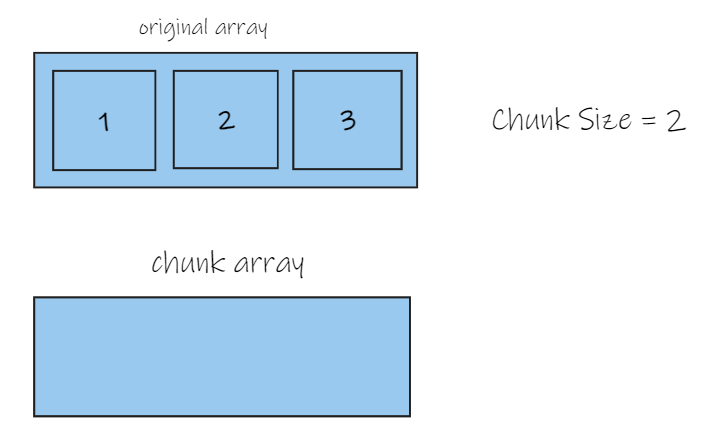
- Iterate over the original array and for each element in the array
- Retrieve the last element in chunk array (remember: NOT THE ORIGINAL ARRAY)
- If last element does exist (or if its length is equal to chunk size), push a new chunk into chunk array
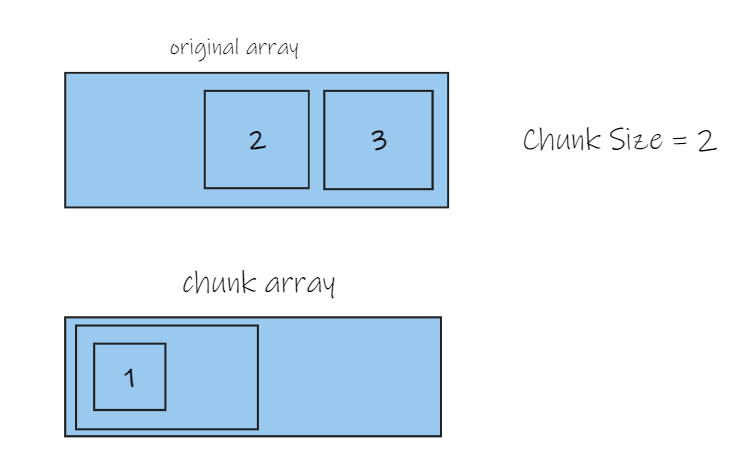
- Else add the current element into the chunk
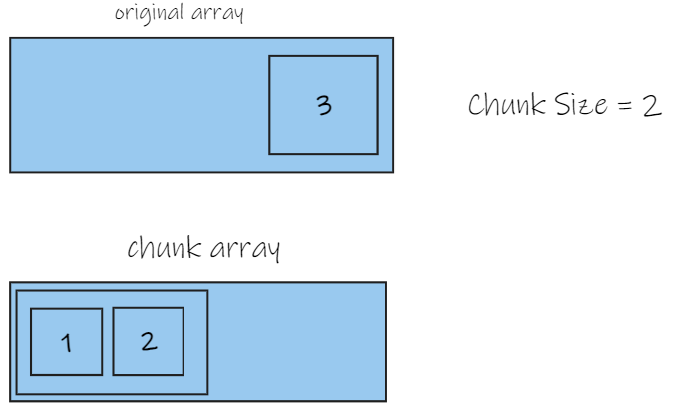
function chunk(array, size) {
//Create an empty array to hold chunk
const chunked = [];
//Iterate thru the original element
for (let element of array) {
//Retrieve last element
const last = chunked[chunked.length - 1]
//If last element does not exist or if its length is equal to chunk size
if(!last || last.length === size) {
//Push a new chunk onto the array
chunked.push([element])
} else {
//Add current element into the chunk
last.push(element);
}
}
return chunked;
}
Chunking with slice
In Javascript, the slice()
method returns a shallow copy of an array from start
to end
(not including the end).
To split an array into two equal chunks, we can use this code:
const chunk1 = arr.slice(0, arr.length / 2);
const chunk2 = arr.slice(arr.length / 2, arr.length);
The first line will contain the first half of the original array and the second line will include the other half. After that, we use a for loop to split the array into multiple chunks of equal size. The loop should iterate over the elements of the original array, using the .slice()
method to extract each chunk and store it in a new array.
function chunkArray(array, size) {
const chunks = [];
for(let i = 0; i < arr.length; i += size) {
const chunk = arr.slice(i, i + size);
chunks.push(chunk);
}
return chunks
}
- First create an array to store the new chunks
- Next, create loop that starts at the size of what we want to chunk
- For each iteration, we slice the array and push the chunk on the new array
Conclusion
Array chunking allows us to create a new array with sub arrays contained inside. Thank you for reading. Happy coding.
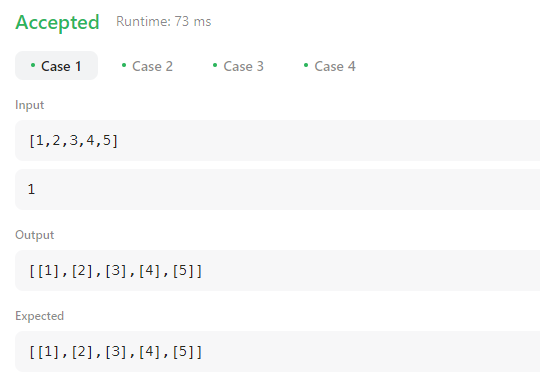