Sorting algorithms have been the subject of massive research in computer science. They all are trying to solve the some problem.
Given an array of unsorted values, how can we sort them so that they end up in ascending order?
Bubble Sort is a sorting algorithm with the following steps:
- Initialize two pointers in the array. One at the first value and the other at the second
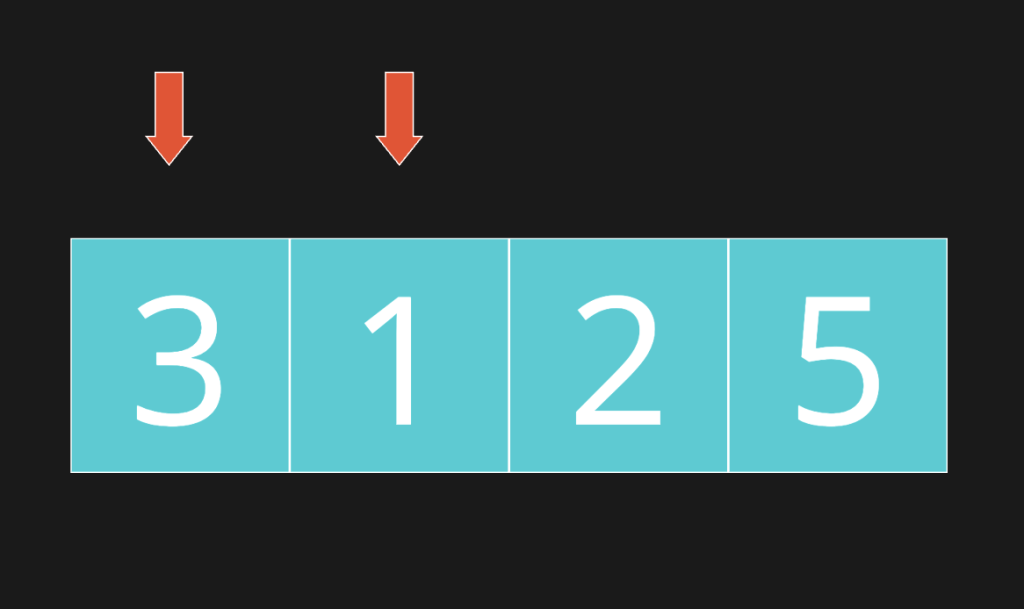
2. If the left value is greater than the right value, swap them. If not, do not swap.
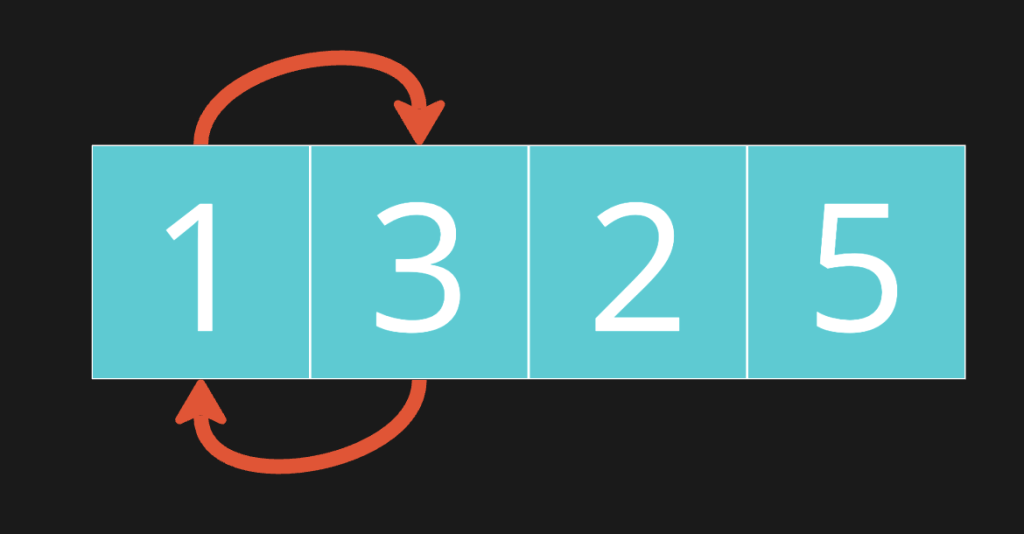
3. Move both pointers to the right.
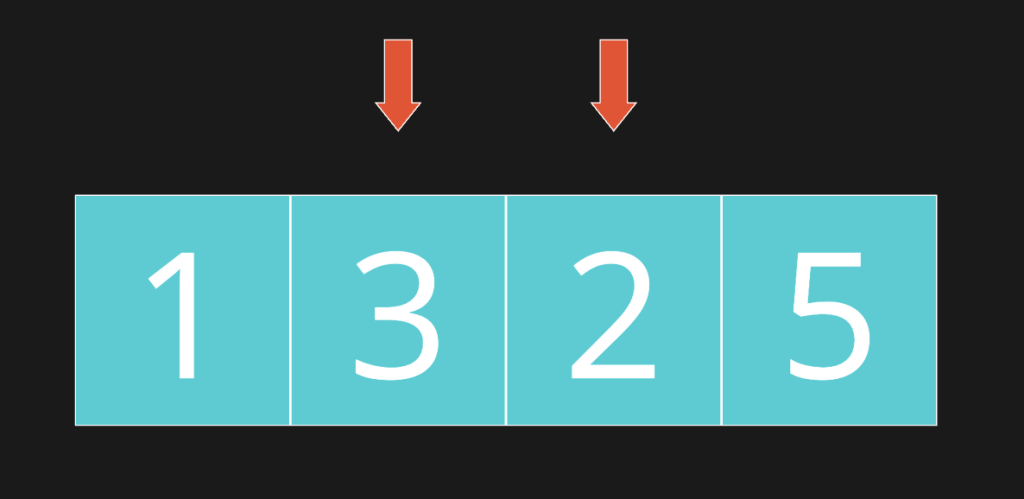
4. Repeat steps 1 through 3 until we reach the end of the array, or if we reach the values that have already been sorted.
5. Move pointers back to the first two values of the array and repeat process 1 through 4 again.
Bubble Sort Implementation
public static int[] BubbleSort(int[] list)
{
int unsortedUntilIndex = list.Length - 1;
bool sorted = false;
while (!sorted)
{
sorted = true;
for (int i = 0; i < unsortedUntilIndex; i++)
{
if (list[i] > list[i + 1])
{
// Swap the elements
int temp = list[i];
list[i] = list[i + 1];
list[i + 1] = temp;
sorted = false;
}
}
unsortedUntilIndex--;
}
return list;
}
- First thing we do is create a variable called
unsortedUntilIndex
. This keeps track of the rightmost index of the array that has not yet been sorted. When we first start the algorithm, the array is not sorted. We need to initilalize this variable to be the final index. - Next we create a boolean called
sorted
.The idea is that in each loop, we’ll assume the array is sorted, until a swap is encountered. If we can get through the entire sort without a swap, then we’ll know for sure the array is sorted. - Create while loop, because we have no idea when we will reach the end of sorting.
- Begin for loop with variables i as first pointer, and it starts from the beginning of array and goes until
unsortedUntilIndex
. - At the end of each pass through, decrement
unsortedUntilIndex
by 1, since the previous index is now sorted.