The title of this LeetCode questions is very ambiguous and does not do a great job explaining what it actually does.
All this interview question is asking you to do is move the zeros to the end of an array. That’s it.
Before:
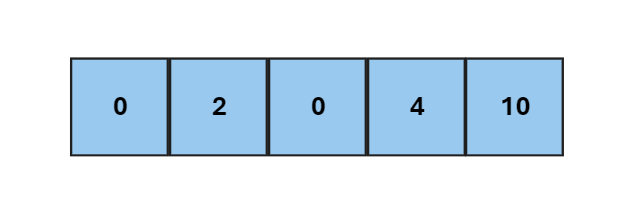
After:
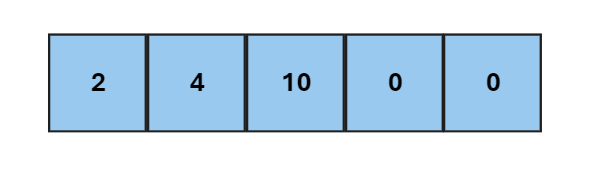
- Key point: The elements don’t have to be sorted. All non-zero elements must retain the original value.
2-Pointer Step-By-Step (In-Place)
- Given that we are required to transform the array in-place, first we create read and write pointers using two pointer method.
- Loop through array
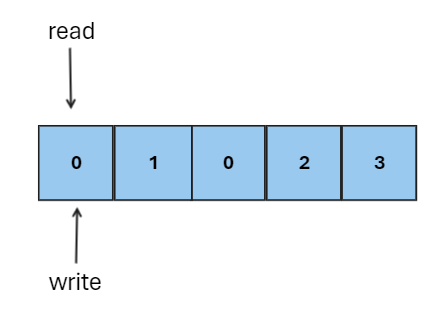
- Check if reader finds a non-zero. If non-zero is found, swap writer with reader value.
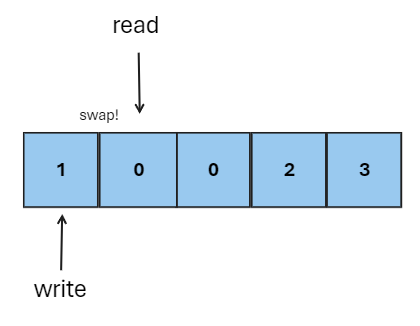
- Increment writer
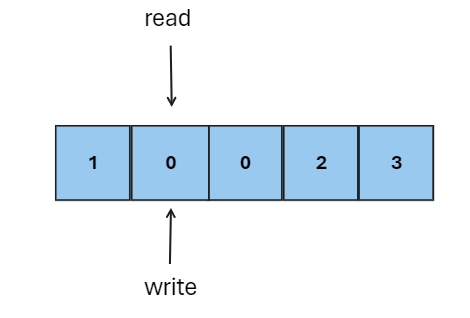
- If zero is found, do not swap, don’t increment writer, and just increment reader
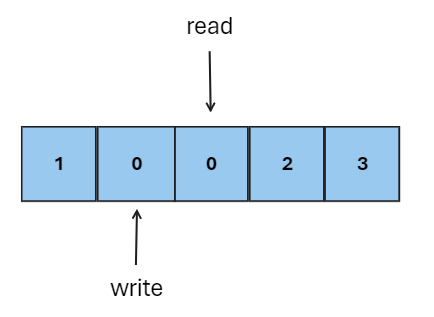
class Solution {
public void moveZeroes(int[] nums) {
//Declare two pointer
int read = 0;
int write = 0;
//Loop thru
while(read < nums.length){
//If non-zero number
if(nums[read] != 0){
//Swap
int temp = nums[reader];
nums[reader] = nums[writer];
nums[writer] = temp;
//Increment write
writer++;
}
//Increment reader regardless
reader++;
}
}
}