Understanding what interfaces are and why we use them can be a real issue when first starting to code.
The real reason we use interfaces is because (like any other programming concept) we are trying to stop duplication.
Interfaces are way better at stopping duplication compared to inheritance; hence why interfaces are everywhere and inheritance is meh.
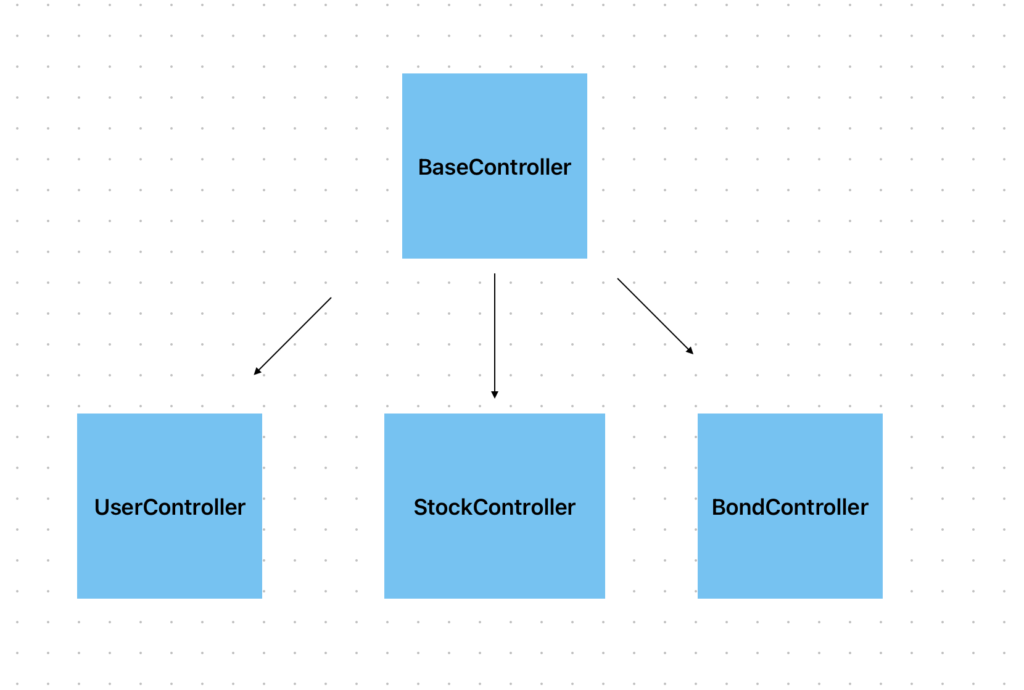
Take for example a BaseController pattern which is very common in .NET and Java. You simply create a BaseClass which then will give all methods to the child classes.
This is great but sometimes we don’t want all those methods, hence why we use interfaces (w/ inheritance for best of both worlds of course! using only interfaces would be silly).
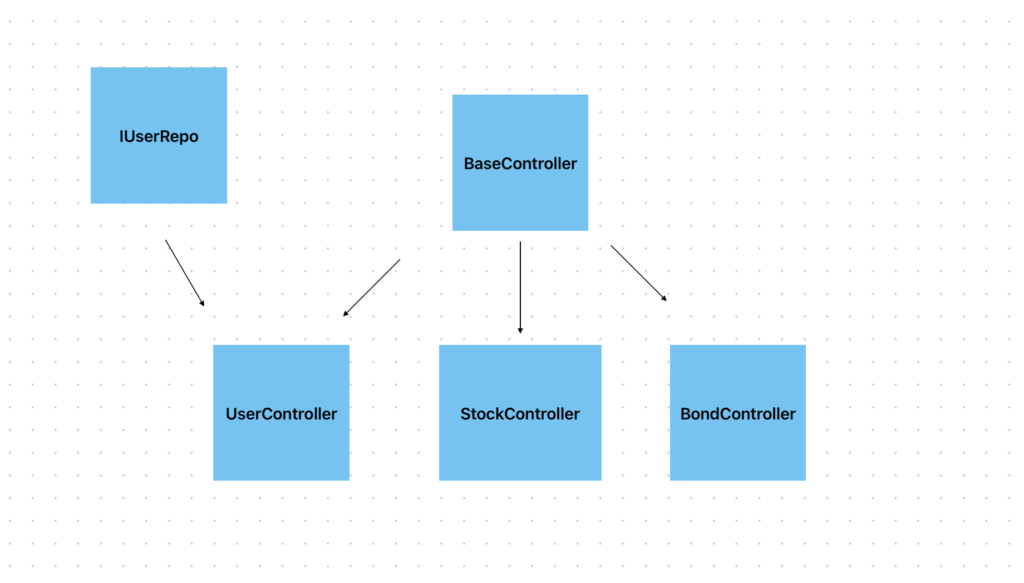
Let’s say we wanted to add some database calls to our UserController. It would make ZERO sense to use inheritance since many of the lower classes don’t need our IUserRepo.
In essence, we are following a very handy design pattern and that is “separate the parts that change from those that stay the same”.
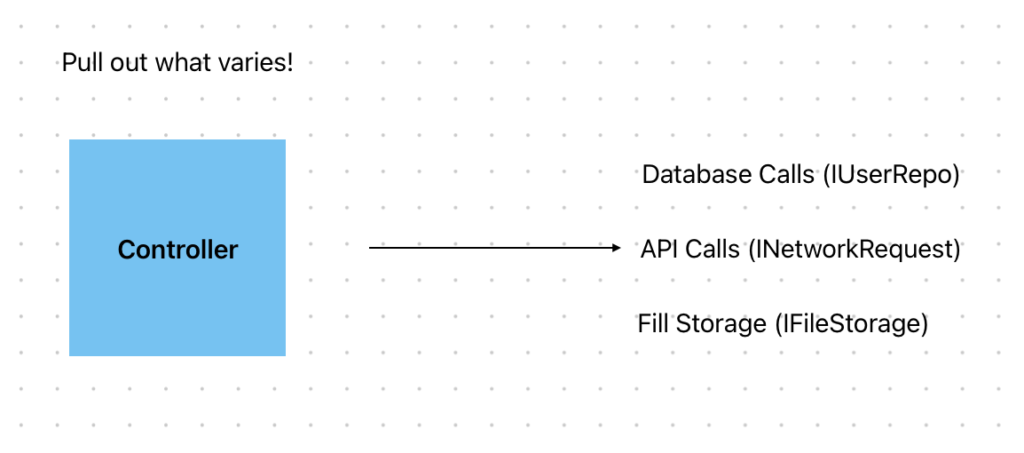
Is-A and Has-A Relationship
Many times developers refer to the above as a is-a and has-a relationship.
If something “is-a” we use inheritance, because in our example a BaseController is-a controller. A BaseController does not technically have a Controller.
A “has-a” relationship is when we use interfaces, because they “have” database calls, network access, file storage, etc.
Conclusion
Interfaces are nothing more then a way to reduce code duplication and move code in our app with more logical seperation. People often times get so carried away learning design patterns without understanding that many times DRY can be achieved with just interfaces.