One-to-many relationships are used when a single entity is associated with many other entities.
For example, a Blog
can have many associated Posts
, BUT each Post
is only associated with one Blog
.
This simply means you map child entities (Posts
) as a collection in the parent object (Blogs
).
Spring Data JPA then provides the @OneToMany annotation for this case. @OneToMany is the parent-side of the relationship. We call this a unidirectional @OneToMany association.
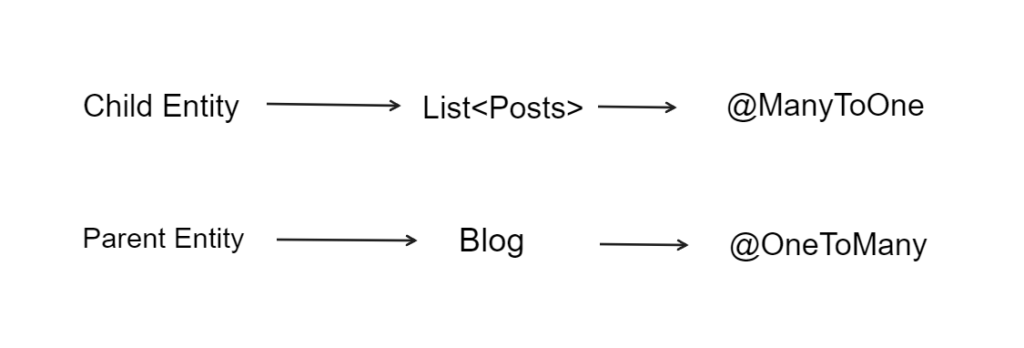
When the child-side manages a relationship, we have a unidirectional @ManyToOne association. In this association, the child entity (Post
) has an object reference to its parent (Blog
).
This child/parent relationship is created by the use of mapping child foreign keys mapping to the parent.
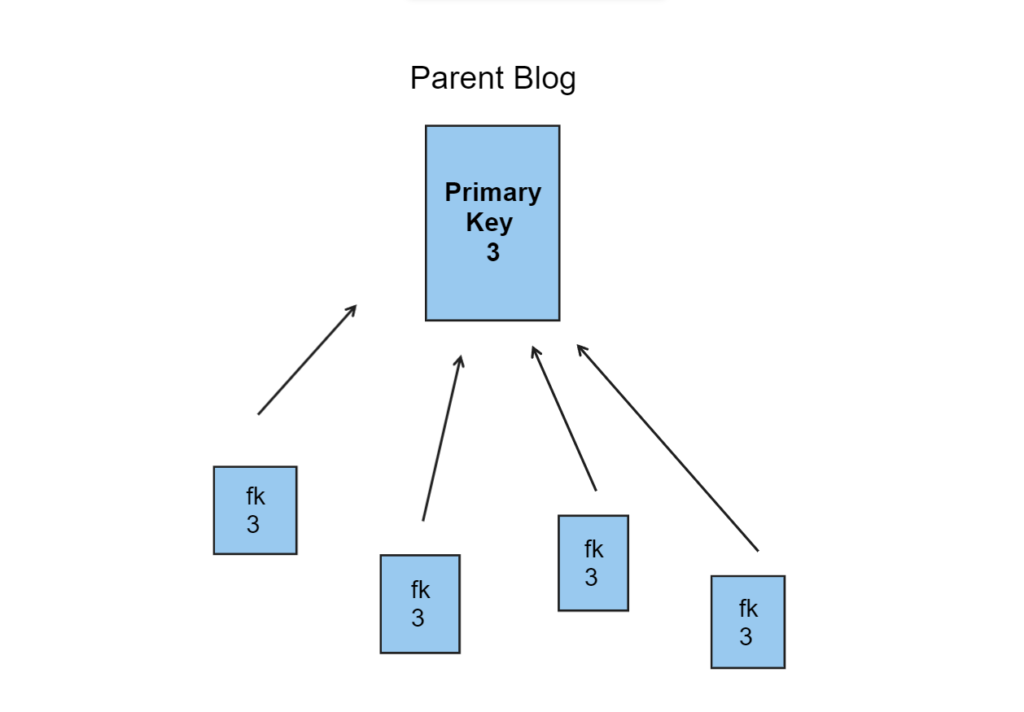
In order to get the most fully functional mapping, it is best to use a unidirectional many-to-one association,
JPA Unidirectional One To Many Example
First, let’s create a model for our Blog model:
@Entity
@Table(name = "blogs")
public class Blog {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private long id;
@Column(name = "title")
private String title;
@Column(name = "description")
private String description;
@Column(name = "published")
private boolean published;
@OneToMany(cascade = CascadeType.ALL, fetch = FetchType.LAZY, orphanRemoval = true)
@JoinColumn(name = "blog_id")
private Set<Comment> comments = new HashSet<>();
}
The above example includes Comments in our OneToMany mapping. We use this annotation in conjunction with @JoinColumn
that will create a column in the child table (Comments
) storing the parent’s Id (Blog
).
The orphanRemoval helps us remove children (Comments
) from the database if we delete the parent (Blog
)
Bidirectional vs Unidirectional Relationships
In our example, we are using a unidirectional relationship. It is important to understand the difference between a unidirectional relationship and bidirectional relationship.
This biggest difference is that bidirectional relationships provide “navigation” access in both directions.
“Navigation” in this sense is a fancy term for, you can access these model properties thru the property.
Navigational access is not always good especially if you have MANY children properties.
Unless you have hundreds or thousands of children, unidirectional is *usually* the best choice. According to Hibernate documentation, bidirectional is the best choice in most cases.
@Entity
@Table(name = "comments")
public class Comment {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "title"
private String title;
@Lob
private String content;
}
Notice in this class we do not need to add any extra annotations because this is a unidirectional relationship.
Pingback:Spring Data JPA Many-To-Many Explained Simply – TeddySmith.IO