If you program long enough, you will make the mistake of “premature optimization”. Premature optimization simply means you put the cart before the horse and made your application too complex. Redux is a framework where this happens quite frequently.
The useReducer hook is a way to manage state in React. There are many ways of handling state and using reducers, but is Redux really necessary?
What are Reducers and Why Do We Need Them?
When you implement any type of state, you have a state variable.
This state updates throughout various stages of your application.
This can become complex.
To combat this, you move all your state updates into a single function.
The function which contains all your state updates is called the reducer. This is because you are reducing all your state updates into a single function
The method you call to perform the operations is the dispatch method.
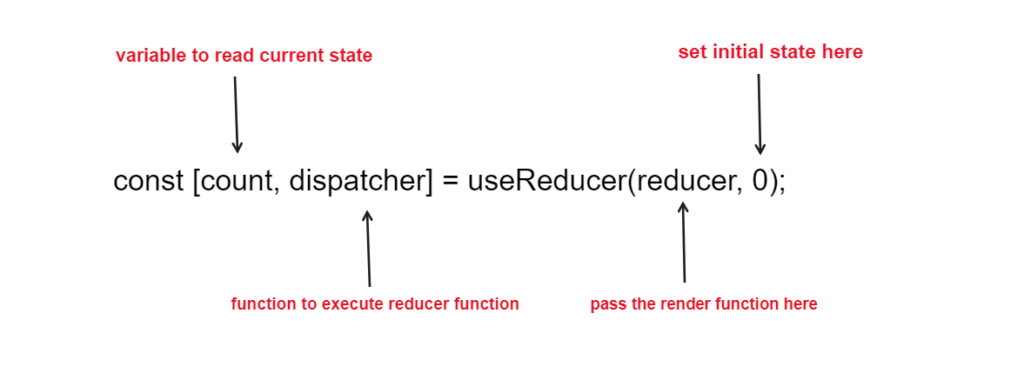
useReducer is similar to useState hook, but it allows you to manage more complex state logix and handle multiple state updates with a reducer function.
To useReducer, you first need to define a reducer function that takes in the current state, an action, and returns a new state.
Then, in your component, you can call useReducer and pass in the reducer and an initial state. This returns an array with the current state and a dispatch method, which can use to trigger state updates by calling the dispatch and passing an action.
import { useReducer } from 'react'
const initialState = {
count: 0,
};
const reducer = (state, action) => {
switch (action.type) {
case "increment":
return { count: state.count + 1 };
case "decrement":
return { count: state.count - 1 };
default:
return state:
}
};
const myComponent = () => {
const [state, dispatch] = useReducer(reducer, intialState);
return (
<>
<h1>Count: {state.count}</h1>
<button onClick={() => dispatch({ type: "increment" })}>INCREMENT</div>
<button onClick={() => dispatch({ type: "decrement" })}>DECREMENT</div>
</>
);
}
Is Redux Necessary Still?
Speaking from experience, I can tell you that Redux can be a huge pain point (especially for new developers).
- If you are a new developer and/or working on a small project, DO NOT USE REDUX.
- If you are an experienced developer and built-in API’s like useReducer are not enough, Redux may be for you.
The biggest reason Redux still makes sense is that it is a global container where everything is held and kept. This means when you are working on a large team, there will be less confusion about where things are.